The puzzle ¶
What is the output of the following piece of code?
public class test {
public static void main(String[] args) {
int i = 1;
i += ++i + i++ + ++i;
int j = 1;
j += ++j + j++ + ++j;
int k = 1;
k += k++ + k++ + ++k;
int m = 1;
System.out.println("i = " + i);
System.out.println("j = " + j);
System.out.println("k = " + k);
System.out.println("m = " + (m += 1));
}
}
. . . . . . . . . . . . . . . . . . . . . .
Answer ¶
The output is:
i = 9
j = 9
k = 8
m = 2
Explanation ¶
Part one ¶
First, take a look at statements of this structure:
i += s
where i
is the integer and s is a statement (e.g. ++i
). This gets evaluated to
i = a + s
Source: docs.oracle.com
Part two ¶
Lets take a look at pre- and postincrement in Java.
You can quite easily figure out what the different increments do by this snippet:
public class test {
public static void main(String[] args) {
int i = 0;
int j = 0;
int k = 0;
System.out.println("i = " + ++i);
System.out.println("i = " + i);
System.out.println("j = " + j++);
System.out.println("j = " + j);
System.out.println("k = " + (k += 1));
}
}
Output:
i = 1
i = 1
j = 0
j = 1
k = 1
Line 7 adds +1 to i
and returns the value.
Line 10 returns the value of j
and adds +1 to j
.
Line 13 adds +1 to k
and returns k
.
Lets return to the original puzzle. Java parses your code from left to right (Source 1, Source 2).
Most important:
Evaluation of an expression can also produce side effects, because expressions may contain embedded assignments, increment operators, decrement operators, and method invocations.
So:
int i = 1;
i += ++i + i++ + ++i;
is the same as
i = ((i + (++i)) + (i++)) + (++i);
The first ++i
increments i
to 2 and returns 2. So you have:
i = 2;
i = ((1 + 2) + (i++)) + (++i);
The i++
returns 2, as it is the new value of i
, and increments i
to 3:
i = 3;
i = ((1 + 2) + 2) + ++i;
The second ++i
increments i
to 4 and returns 4:
i = 4;
i += ((1 + 2) + 2) + 4;
So you end up with 9
.
A parse tree of this evaluation would look like this:
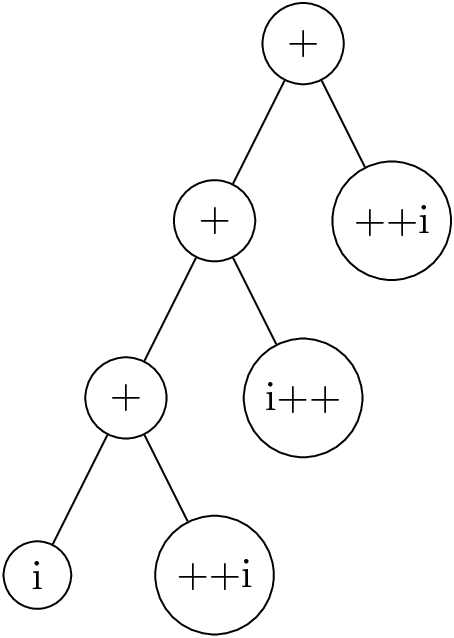
The explanation for the other three ones is similar.